Most of my coding experience has been with JavaScript and JavaScript frameworks. So, I’ve been thinking recently about how I can keep my knowledge of JavaScript current and not get too rusty in this post-bootcamp, pre-job period of my life. Today, I opted to do some coding challenges on Codewars to stay fresh.
Codewars is a great tool for practicing solving coding challenges on your own. And I used Codewars throughout my bootcamp experience to practice what I had been learning and hopefully prepare for coding challenges in interview situations.
Almost every time I sit down to do a coding challenge, I’m struck with one of two emotions: joy or utter discouragement. Joy happens when I work through a challenge, put it all together, run the tests, and it passes. Woo hoo! I sort of know what I’m doing! Discouragement, on the other hand, happens when I work through a challenge, put it all together, work through errors or mistakes, and I just can’t make it pass the tests.
Thankfully, today, my experience was one of joy, not discouragement. Yay! But, I was also faced with the slap in the face that comes when you look at alternative solutions. Duh! Why didn’t I think of that? Of course! And today, omg. That’s a thing?!
I’ve written about what it feels like to be a novice for me, and a part of that includes constantly coming face-to-face with things you don’t know yet. Today, one of those things for me was the .endsWith() method in JavaScript.
So, in this post, I want to share my solution for the coding challenge I put together initially, and then I’ll explore the .endsWith() method a bit. Because, yes, it’s a thing.
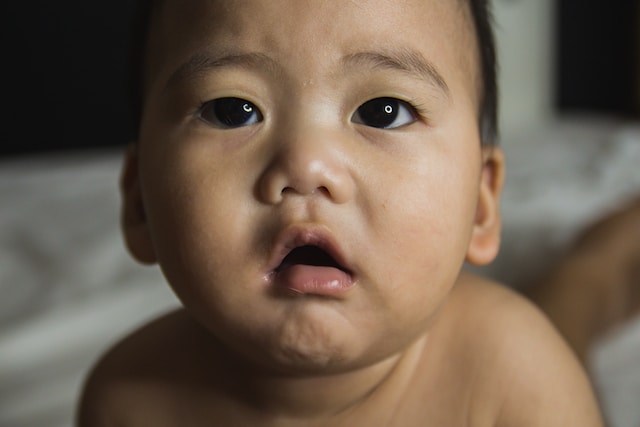
String ends with?
The kata I was tasked with today was called “String ends with?”, and it was a 7kyu level. Which means, it’s pretty simple. I wanted to practice the simple stuff, make sure I haven’t totally lost all of the things I’ve learned about JavaScript basics. So this 7kyu seemed perfect.
The challenge read:
Complete the solution so that it returns true if the first argument(string) passed in ends with the 2nd argument (also a string).
Examples:
solution(‘abc’, ‘bc’) // returns true
solution(‘abc’, ‘d’) // returns false
It seemed like something I could get into, some basic string manipulation. Ok, so my initial approach was to loop through the string, compare it to the other string, and return true if they matched (on the ending). To do this, I worked out this pseudocode:
- Reverse str
- Reverse ending
- Loop over str
- If str = ending, push to new array
- Make array back into a string
- Make ending back into a string
- If array = reversed ending, true
- If array ≠ reversed ending, false
I thought reversing the input would allow me to more easily compare the characters in the loops, so it would start at the beginning (the end) and I wouldn’t need to specify which characters to compare otherwise.
Cool. So, this is the function that I built out to solve this coding challenge:
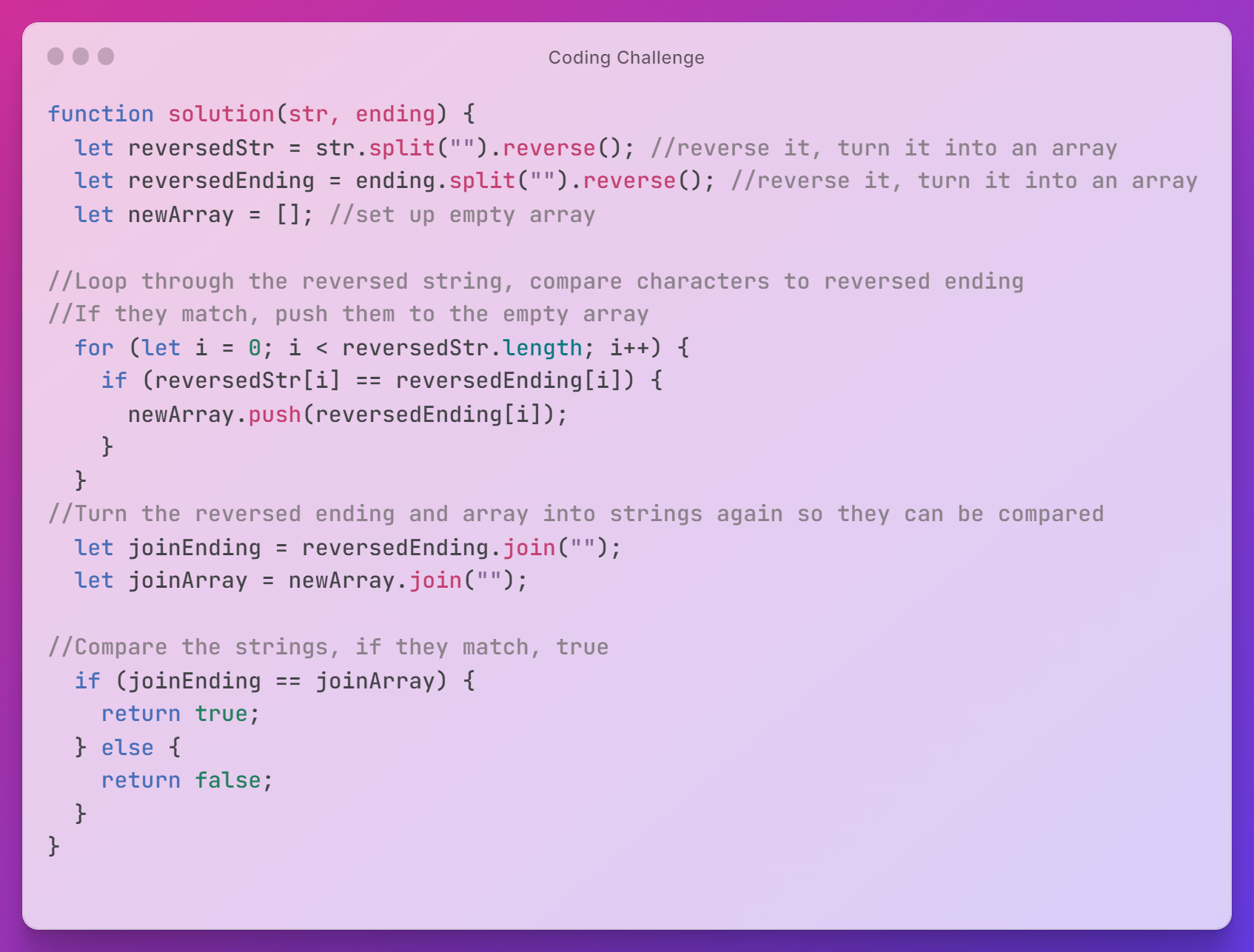
And it works. But it’s kind of long, and you know, I submitted it knowing there were probably really clever ways of solving this challenge that I hadn’t thought of. But, I was not prepared to see the SUPER simple solution that involved just one method.
Enter: .endsWith( )
.endsWith( ) JavaScript Method
The .endsWith( ) JavaScript method is a built-in method that returns true if a string ends with a specified string. It returns false if it doesn’t. It’s case-sensitive. And it’s perfect for this coding challenge.
But, I didn’t know it was a thing. And, apparently, .startsWith( ) is also a thing. Put these little baby methods in your memory bank for later.
So, my new solution to the coding challenge is this little angel:
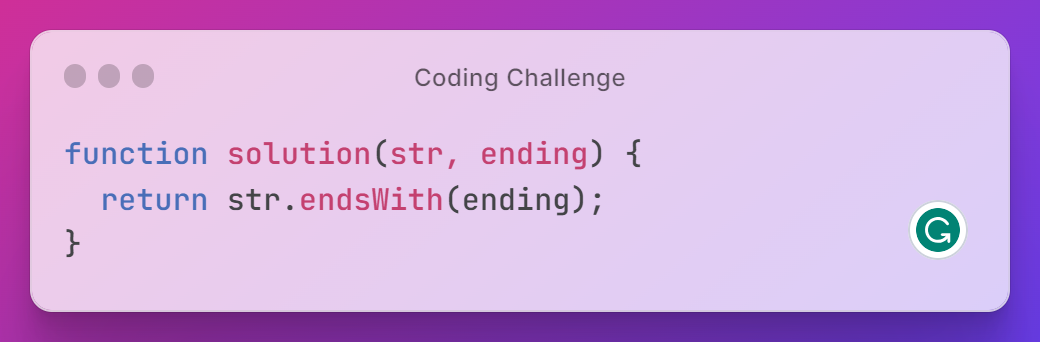
The moral of this story is that there’s always something new to learn. And there are multiple ways to solve any given challenge.